Your cart is currently empty!
A Beginner’s Guide to Programming with NVIDIA CUDA
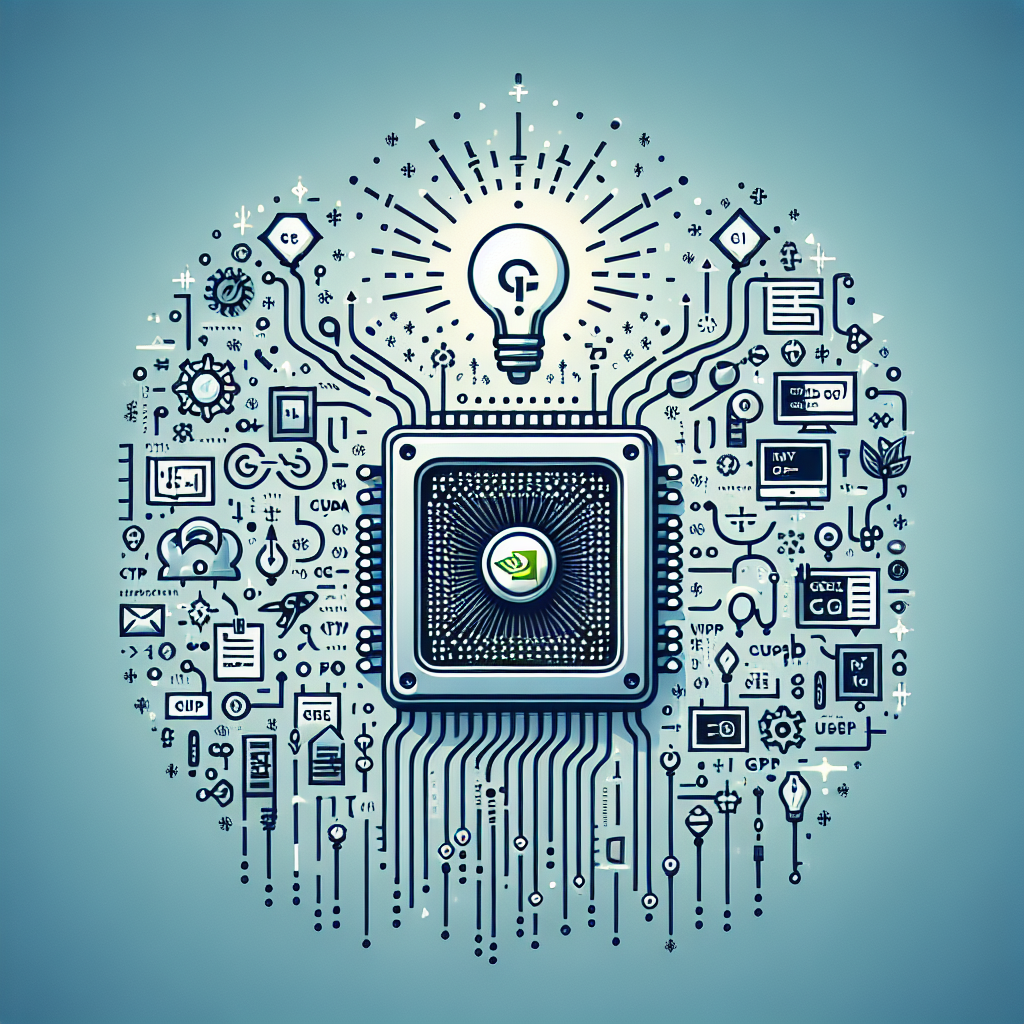
If you’re interested in diving into the world of parallel computing and accelerating your programs using GPU technology, NVIDIA CUDA is a great place to start. CUDA, which stands for Compute Unified Device Architecture, is a parallel computing platform and programming model that allows developers to harness the power of NVIDIA GPUs for general-purpose computing.
In this beginner’s guide, we’ll cover the basics of programming with CUDA and provide you with the tools you need to get started.
Getting Started with CUDA
To begin programming with CUDA, you’ll need a computer with an NVIDIA GPU that supports CUDA. You can check the list of supported GPUs on NVIDIA’s website. You’ll also need to install the CUDA Toolkit, which includes the necessary libraries, tools, and compilers for developing CUDA applications.
Once you have the CUDA Toolkit installed, you can start writing CUDA code using the CUDA C programming language. CUDA C is an extension of the C programming language that includes special keywords and constructs for parallel programming on NVIDIA GPUs.
Writing Your First CUDA Program
To get started with CUDA programming, let’s write a simple program that adds two arrays together on the GPU. Here’s a basic outline of the code:
“`c
#include
__global__ void add(int *a, int *b, int *c, int n) {
int i = blockIdx.x * blockDim.x + threadIdx.x;
if (i < n) { c[i] = a[i] + b[i]; } } int main() { int n = 100; int a[n], b[n], c[n]; int *dev_a, *dev_b, *dev_c; cudaMalloc((void**)&dev_a, n * sizeof(int)); cudaMalloc((void**)&dev_b, n * sizeof(int)); cudaMalloc((void**)&dev_c, n * sizeof(int)); // Initialize arrays a and b for (int i = 0; i < n; i++) { a[i] = i; b[i] = i * 2; } cudaMemcpy(dev_a, a, n * sizeof(int), cudaMemcpyHostToDevice); cudaMemcpy(dev_b, b, n * sizeof(int), cudaMemcpyHostToDevice); add<<<1, n>>>(dev_a, dev_b, dev_c, n);
cudaMemcpy(c, dev_c, n * sizeof(int), cudaMemcpyDeviceToHost);
for (int i = 0; i < n; i++) { printf(“%d + %d = %d\n”, a[i], b[i], c[i]); } cudaFree(dev_a); cudaFree(dev_b); cudaFree(dev_c); return 0; } “` In this code, we define a kernel function `add` that adds two arrays `a` and `b` together and stores the result in array `c`. We then allocate memory on the GPU for the arrays using `cudaMalloc` and copy the data from the host to the device using `cudaMemcpy`. We launch the kernel using the `<<<1, n>>>` syntax, which specifies that we want to use one block with `n` threads. Finally, we copy the result back to the host and print the output.
Compile and Run Your CUDA Program
To compile the CUDA program, you’ll need to use the `nvcc` compiler that comes with the CUDA Toolkit. You can compile the program by running the following command in the terminal:
“`bash
nvcc your_program.cu -o your_program
“`
And then run the compiled program:
“`bash
./your_program
“`
Congratulations! You’ve just written and run your first CUDA program.
Conclusion
In this beginner’s guide, we covered the basics of programming with NVIDIA CUDA and wrote a simple CUDA program that adds two arrays together on the GPU. As you continue to explore CUDA programming, you’ll discover the power and performance benefits of using GPUs for parallel computing tasks. Happy coding!
Leave a Reply