Your cart is currently empty!
Building a Deep Learning Model with LSTMs: A Step-by-Step Tutorial
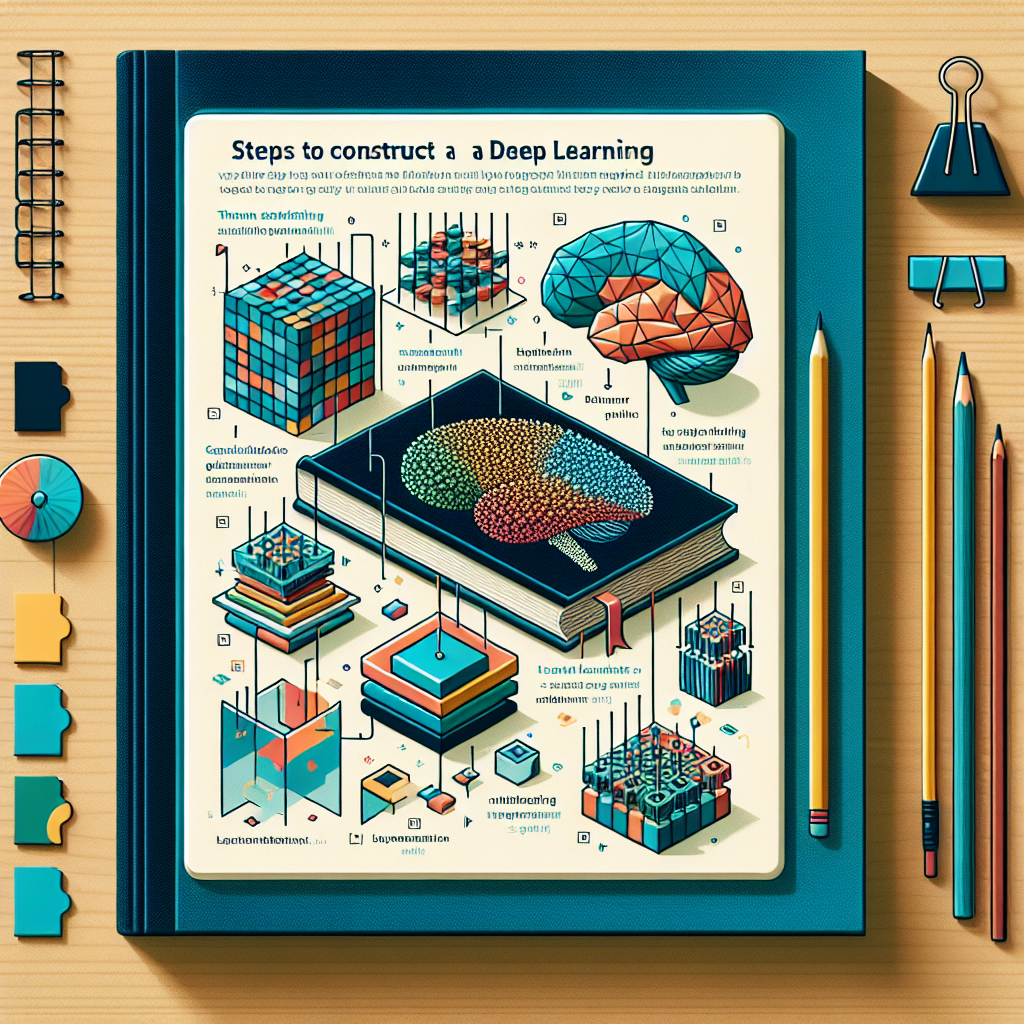
Deep learning models have become increasingly popular in the field of artificial intelligence, particularly in applications that involve processing and analyzing sequential data. Long Short-Term Memory (LSTM) networks are a type of deep learning model that is well-suited for sequential data, making them an ideal choice for tasks such as natural language processing, time series analysis, and speech recognition.
In this tutorial, we will walk through the process of building a deep learning model with LSTMs using Python and the Keras library. By the end of this tutorial, you will have a deep understanding of how LSTMs work and how to implement them in your own projects.
Step 1: Import the necessary libraries
The first step in building our LSTM model is to import the necessary libraries. We will be using numpy for numerical operations, pandas for data manipulation, and Keras for building and training our deep learning model.
“`python
import numpy as np
import pandas as pd
from keras.models import Sequential
from keras.layers import LSTM, Dense
“`
Step 2: Load and preprocess the data
For this tutorial, we will be using a simple dataset containing time series data. You can use any dataset of your choice, as long as it is in a format that can be easily loaded into a pandas DataFrame.
“`python
data = pd.read_csv(‘data.csv’)
“`
Next, we need to preprocess the data by normalizing it and splitting it into input and output sequences. This step is crucial for training our LSTM model effectively.
“`python
data = (data – data.min()) / (data.max() – data.min())
X = data.iloc[:, :-1].values
y = data.iloc[:, -1].values
“`
Step 3: Reshape the input data
LSTMs require input data to be in a specific format: a 3D array with dimensions [batch_size, time_steps, input_dim]. To achieve this, we need to reshape our input data accordingly.
“`python
X = np.reshape(X, (X.shape[0], X.shape[1], 1))
“`
Step 4: Build the LSTM model
Now that we have preprocessed our data, we can proceed to build our LSTM model. We will create a sequential model and add an LSTM layer with 50 units, followed by a dense output layer with one unit.
“`python
model = Sequential()
model.add(LSTM(units=50, input_shape=(X.shape[1], 1)))
model.add(Dense(units=1))
“`
Step 5: Compile and train the model
Before training our model, we need to compile it with an appropriate loss function and optimizer. For this tutorial, we will use the mean squared error loss function and the Adam optimizer.
“`python
model.compile(optimizer=’adam’, loss=’mean_squared_error’)
model.fit(X, y, epochs=100, batch_size=32)
“`
Step 6: Make predictions
Once our model has been trained, we can use it to make predictions on new data. We can do this by reshaping the input data and calling the predict method on our model.
“`python
new_data = np.array([[0.1, 0.2, 0.3]])
new_data = np.reshape(new_data, (1, new_data.shape[1], 1))
prediction = model.predict(new_data)
print(prediction)
“`
In this tutorial, we have covered the basic steps involved in building a deep learning model with LSTMs. By following these steps, you can create your own LSTM models for a variety of sequential data tasks. Remember to experiment with different architectures, hyperparameters, and datasets to optimize the performance of your model.
#Building #Deep #Learning #Model #LSTMs #StepbyStep #Tutorial,lstm
Leave a Reply