Your cart is currently empty!
Demystifying Deep Learning: A Beginner’s Guide to Building Machine Learning Systems with PyTorch and TensorFlow
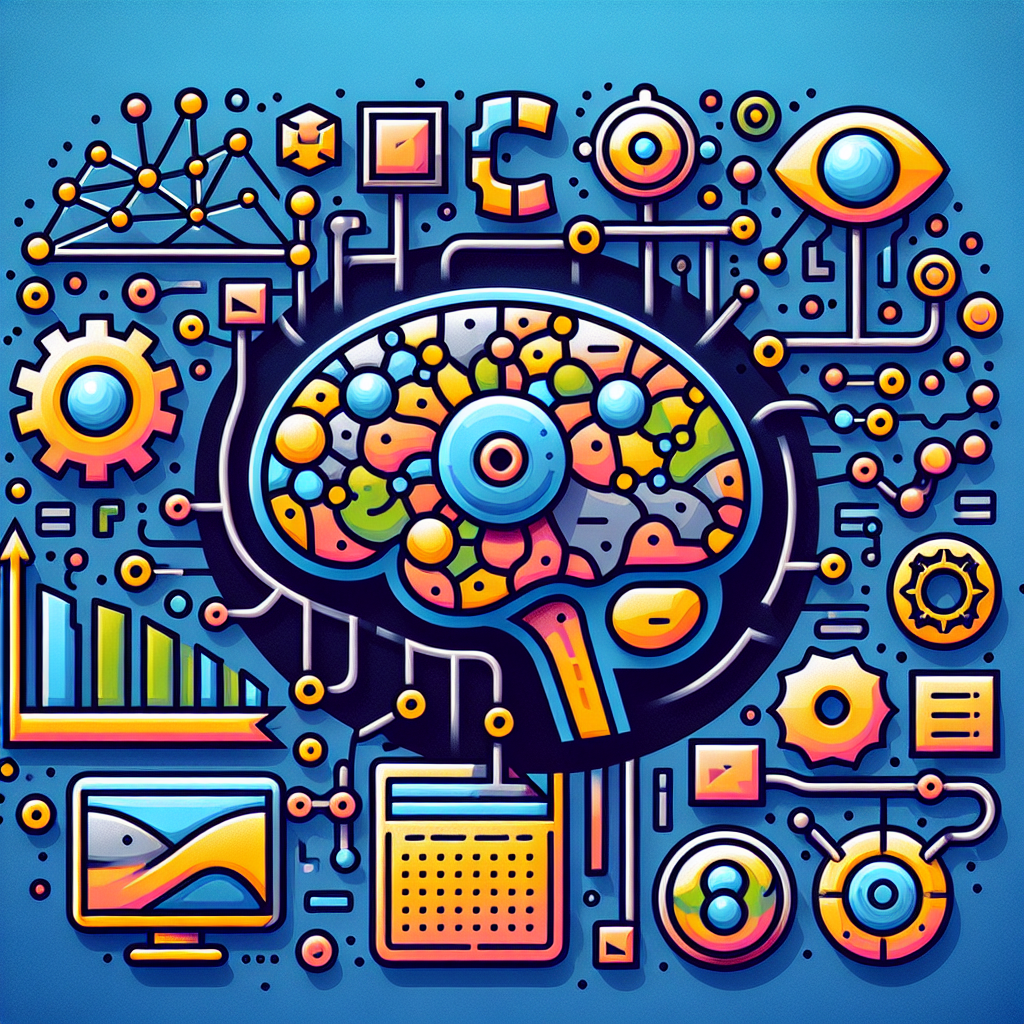
Deep learning has become one of the most popular and powerful techniques in the field of artificial intelligence. It has revolutionized the way we approach machine learning tasks, allowing us to tackle complex problems with unprecedented accuracy and efficiency. However, for beginners, deep learning can seem like a daunting and mysterious field.
In this article, we will demystify deep learning and provide a beginner’s guide to building machine learning systems with two of the most popular deep learning frameworks: PyTorch and TensorFlow.
What is Deep Learning?
Deep learning is a subset of machine learning that focuses on neural networks – algorithms inspired by the structure and function of the human brain. These neural networks are composed of layers of interconnected nodes, each performing a specific mathematical operation on the input data. By stacking multiple layers together, deep learning models can learn complex patterns and relationships in the data, making them incredibly powerful for tasks such as image recognition, natural language processing, and speech recognition.
PyTorch: A Flexible and Dynamic Framework
PyTorch is a deep learning framework developed by Facebook’s AI Research lab. It is known for its flexibility and dynamic computation graph, which allows for easy experimentation and rapid prototyping. PyTorch’s Pythonic syntax and intuitive API make it a popular choice among researchers and practitioners alike.
To build a machine learning system with PyTorch, you first need to install the framework and its dependencies. You can do this using the pip package manager:
“`
pip install torch torchvision
“`
Once you have PyTorch installed, you can start building your deep learning models. Here’s a simple example of how to create a neural network using PyTorch:
“`python
import torch
import torch.nn as nn
import torch.optim as optim
# Define the neural network architecture
class NeuralNetwork(nn.Module):
def __init__(self):
super(NeuralNetwork, self).__init__()
self.fc1 = nn.Linear(784, 128)
self.fc2 = nn.Linear(128, 10)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
# Create an instance of the neural network
model = NeuralNetwork()
# Define the loss function and optimizer
criterion = nn.CrossEntropyLoss()
optimizer = optim.SGD(model.parameters(), lr=0.01)
# Train the model
for epoch in range(num_epochs):
for inputs, labels in dataloader:
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
“`
TensorFlow: A Powerful and Scalable Framework
TensorFlow is another popular deep learning framework developed by Google. It is known for its scalability and distributed computing capabilities, making it ideal for training large models on massive datasets. TensorFlow’s static computation graph and high-level APIs make it a great choice for production-grade machine learning systems.
To build a machine learning system with TensorFlow, you first need to install the framework and its dependencies. You can do this using the pip package manager:
“`
pip install tensorflow
“`
Once you have TensorFlow installed, you can start building your deep learning models. Here’s a simple example of how to create a neural network using TensorFlow’s Keras API:
“`python
import tensorflow as tf
from tensorflow.keras import layers
# Define the neural network architecture
model = tf.keras.Sequential([
layers.Dense(128, activation=’relu’, input_shape=(784,)),
layers.Dense(10)
])
# Compile the model
model.compile(optimizer=’sgd’, loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True))
# Train the model
model.fit(train_dataset, epochs=num_epochs)
“`
Conclusion
In this article, we have demystified deep learning and provided a beginner’s guide to building machine learning systems with PyTorch and TensorFlow. By following the examples and guidelines outlined in this article, you can start your journey into the exciting world of deep learning and harness its power to solve complex problems and drive innovation in the field of artificial intelligence.
#Demystifying #Deep #Learning #Beginners #Guide #Building #Machine #Learning #Systems #PyTorch #TensorFlow,understanding deep learning: building machine learning systems with pytorch
and tensorflow: from neural networks (cnn
Leave a Reply