Your cart is currently empty!
Exploring Data Analytics with Generative AI in Python: A Step-by-Step Tutorial
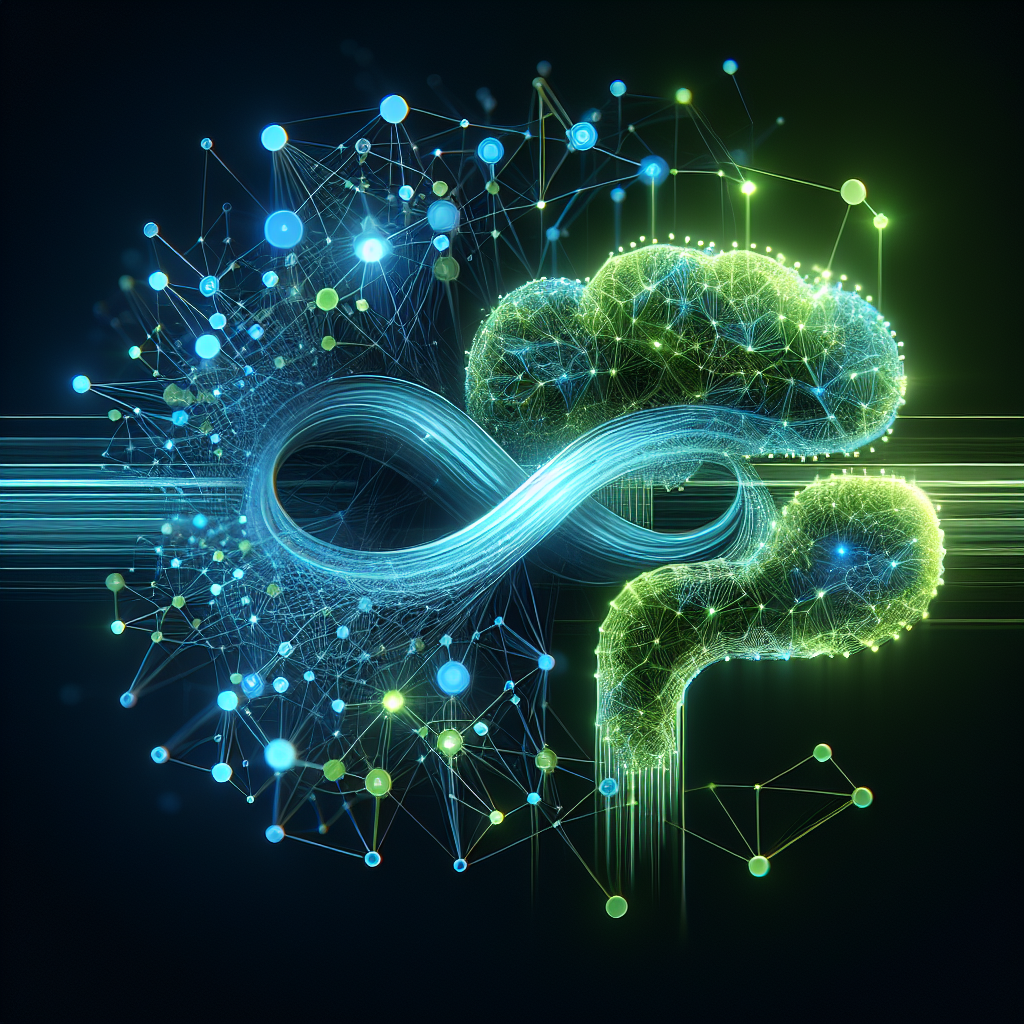
Data analytics is a powerful tool that can help businesses make informed decisions based on patterns and trends in their data. However, traditional data analytics methods can be limited in their ability to generate new insights from data. This is where generative artificial intelligence (AI) comes in.
Generative AI is a branch of artificial intelligence that focuses on creating new data based on patterns in existing data. By using generative AI algorithms, businesses can uncover hidden patterns in their data and generate new insights that can help drive decision-making.
In this step-by-step tutorial, we will explore how to use generative AI in Python to analyze data and generate new insights. By following this tutorial, you will learn how to leverage the power of generative AI to uncover hidden patterns in your data and make more informed decisions.
Step 1: Install the necessary libraries
To get started with generative AI in Python, you will need to install the necessary libraries. The most popular library for generative AI in Python is TensorFlow, which is an open-source machine learning library developed by Google. You can install TensorFlow by running the following command in your terminal:
pip install tensorflow
Step 2: Load and preprocess the data
Next, you will need to load and preprocess the data that you want to analyze. For this tutorial, we will use a sample dataset of customer purchase history. You can load the data using pandas, which is a popular data manipulation library in Python. Here’s an example code snippet to load the data:
import pandas as pd
data = pd.read_csv(‘customer_purchase_history.csv’)
Step 3: Train a generative AI model
Now that you have loaded the data, you can train a generative AI model to analyze the data and generate new insights. In this tutorial, we will use a generative adversarial network (GAN), which is a popular generative AI model. You can train a GAN model using TensorFlow by following the code snippet below:
import tensorflow as tf
from tensorflow.keras.layers import Dense, LeakyReLU, Dropout
from tensorflow.keras.models import Sequential
# Define the generator model
generator = Sequential([
Dense(128, input_shape=(100,)),
LeakyReLU(alpha=0.01),
Dropout(0.3),
Dense(256),
LeakyReLU(alpha=0.01),
Dropout(0.3),
Dense(512),
LeakyReLU(alpha=0.01),
Dense(784, activation=’sigmoid’)
])
# Define the discriminator model
discriminator = Sequential([
Dense(512, input_shape=(784,)),
LeakyReLU(alpha=0.01),
Dropout(0.3),
Dense(256),
LeakyReLU(alpha=0.01),
Dropout(0.3),
Dense(128),
LeakyReLU(alpha=0.01),
Dense(1, activation=’sigmoid’)
])
# Compile the models
generator.compile(loss=’binary_crossentropy’, optimizer=’adam’)
discriminator.compile(loss=’binary_crossentropy’, optimizer=’adam’)
# Combine the models
discriminator.trainable = False
gan = Sequential([generator, discriminator])
gan.compile(loss=’binary_crossentropy’, optimizer=’adam’)
# Train the GAN model
for epoch in range(10000):
noise = np.random.normal(0, 1, (batch_size, 100))
fake_data = generator.predict(noise)
real_data = data.sample(batch_size)
X = np.concatenate((real_data, fake_data))
y = np.concatenate((np.ones((batch_size, 1)), np.zeros((batch_size, 1))))
d_loss = discriminator.train_on_batch(X, y)
noise = np.random.normal(0, 1, (batch_size, 100))
y = np.ones((batch_size, 1))
a_loss = gan.train_on_batch(noise, y)
print(f’Epoch: {epoch}, Discriminator Loss: {d_loss}, Adversarial Loss: {a_loss}’)
Step 4: Generate new insights
Once you have trained the generative AI model, you can use it to generate new insights from your data. For example, you can use the model to generate new customer purchase histories based on the patterns in the existing data. By analyzing these generated insights, you can uncover hidden patterns and trends that can help drive decision-making in your business.
In conclusion, generative AI is a powerful tool that can help businesses uncover hidden patterns in their data and generate new insights. By following this step-by-step tutorial, you can learn how to use generative AI in Python to analyze data and make more informed decisions. So, why not give it a try and explore the world of data analytics with generative AI today?
#Exploring #Data #Analytics #Generative #Python #StepbyStep #Tutorial,starting data analytics with generative ai and python
Leave a Reply