Your cart is currently empty!
Mastering Neural Networks: How to Develop CNN Models with PyTorch and TensorFlow
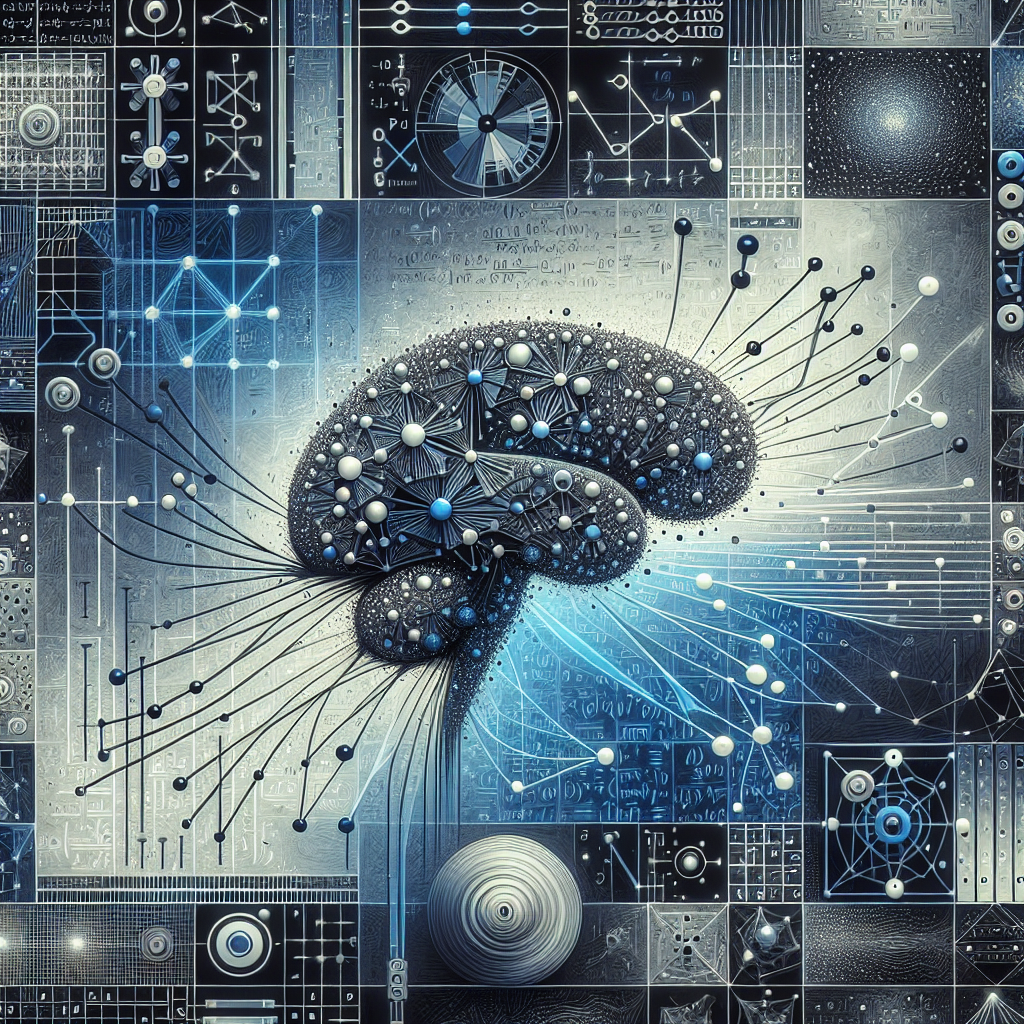
Neural networks have revolutionized the field of artificial intelligence and machine learning. Convolutional Neural Networks (CNN) in particular have been instrumental in advancing computer vision tasks such as image recognition and object detection. If you are looking to master the art of developing CNN models using popular frameworks like PyTorch and TensorFlow, you’re in the right place.
PyTorch and TensorFlow are two of the most widely used deep learning frameworks in the industry. They offer a comprehensive suite of tools and libraries for building, training, and deploying neural networks. In this article, we will walk you through the process of developing CNN models using both PyTorch and TensorFlow.
To start with, let’s understand the basics of CNNs. A CNN is a type of neural network that is specifically designed for processing grid-like data, such as images. It consists of multiple layers of convolutional and pooling operations, followed by fully connected layers for classification. The convolutional layers learn to extract relevant features from the input data, while the pooling layers reduce the spatial dimensions of the feature maps.
In PyTorch, you can define a CNN model using the nn.Module class. Here’s an example of a simple CNN model in PyTorch:
“`
import torch
import torch.nn as nn
class CNNModel(nn.Module):
def __init__(self):
super(CNNModel, self).__init__()
self.conv1 = nn.Conv2d(in_channels=1, out_channels=16, kernel_size=3, stride=1, padding=1)
self.pool = nn.MaxPool2d(kernel_size=2, stride=2)
self.fc1 = nn.Linear(16 * 14 * 14, 10)
def forward(self, x):
x = self.conv1(x)
x = self.pool(x)
x = x.view(-1, 16 * 14 * 14)
x = self.fc1(x)
return x
“`
In this model, we have defined a convolutional layer with 16 output channels, followed by a max pooling layer and a fully connected layer for classification. To train the model, you can use the PyTorch’s DataLoader class to load and preprocess the data, and the nn.CrossEntropyLoss class for the loss function.
Similarly, in TensorFlow, you can define a CNN model using the tf.keras.Sequential class. Here’s an example of a CNN model in TensorFlow:
“`
import tensorflow as tf
from tensorflow.keras import layers
model = tf.keras.Sequential([
layers.Conv2D(filters=16, kernel_size=3, activation=’relu’, input_shape=(28, 28, 1)),
layers.MaxPooling2D(pool_size=2),
layers.Flatten(),
layers.Dense(10, activation=’softmax’)
])
“`
In this model, we have defined a convolutional layer with 16 filters, followed by a max pooling layer, a flatten layer, and a fully connected layer for classification. To train the model, you can use the tf.keras.optimizers.Adam class for optimization and the tf.losses.SparseCategoricalCrossentropy class for the loss function.
To improve the performance of your CNN models, you can experiment with different architectures, hyperparameters, and optimization techniques. You can also use pre-trained models or transfer learning to leverage the knowledge learned from large datasets.
In conclusion, mastering neural networks and developing CNN models with frameworks like PyTorch and TensorFlow requires practice, experimentation, and a deep understanding of the underlying principles. By following the guidelines provided in this article and exploring the vast resources available online, you can become proficient in building state-of-the-art CNN models for a wide range of computer vision tasks.
#Mastering #Neural #Networks #Develop #CNN #Models #PyTorch #TensorFlow,understanding deep learning: building machine learning systems with pytorch
and tensorflow: from neural networks (cnn
Leave a Reply