Your cart is currently empty!
TensorFlow Reinforcement Learning Quick Start Guide: Get up and running with training and deploying intelligent, self-learning agents using Python
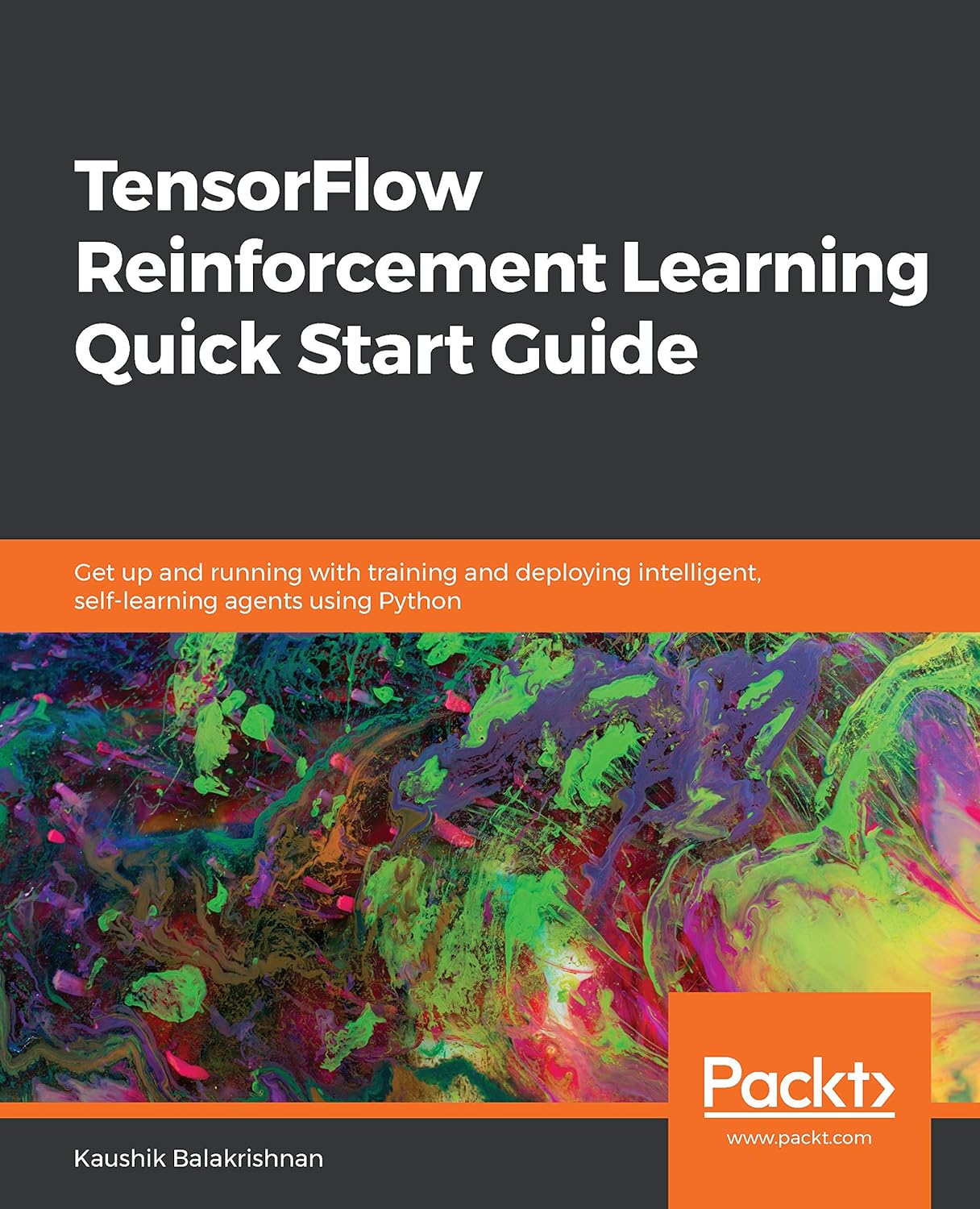
Price: $43.99
(as of Dec 25,2024 01:27:06 UTC – Details)
ASIN : B07Q98P33S
Publisher : Packt Publishing; 1st edition (March 30, 2019)
Publication date : March 30, 2019
Language : English
File size : 4411 KB
Text-to-Speech : Enabled
Screen Reader : Supported
Enhanced typesetting : Enabled
X-Ray : Not Enabled
Word Wise : Not Enabled
Print length : 186 pages
Are you interested in diving into the world of reinforcement learning with TensorFlow? Look no further! In this quick start guide, we will walk you through the process of training and deploying intelligent, self-learning agents using Python.
First, let’s make sure you have TensorFlow installed on your machine. You can install it using pip by running the following command:
pip install tensorflow<br />
```<br />
<br />
Next, you'll need to install other necessary libraries such as gym, a popular toolkit for developing and comparing reinforcement learning algorithms. You can install it using the following command:<br />
<br />
```bash<br />
pip install gym<br />
```<br />
<br />
Now that you have all the necessary libraries installed, let's get started with training your first reinforcement learning agent. We'll start by creating a simple environment using gym. Here's an example of creating a CartPole environment:<br />
<br />
```python<br />
import gym<br />
<br />
env = gym.make('CartPole-v1')<br />
```<br />
<br />
Next, we'll create a neural network model using TensorFlow to act as our agent. Here's an example of a simple neural network model using TensorFlow's Keras API:<br />
<br />
```python<br />
import tensorflow as tf<br />
from tensorflow import keras<br />
<br />
model = keras.Sequential([<br />
keras.layers.Dense(128, activation='relu', input_shape=(env.observation_space.shape[0],)),<br />
keras.layers.Dense(64, activation='relu'),<br />
keras.layers.Dense(env.action_space.n, activation='linear')<br />
])<br />
```<br />
<br />
Now, we can train our agent using a reinforcement learning algorithm such as Deep Q-Learning. Here's an example of training the agent:<br />
<br />
```python<br />
from tensorflow.keras.optimizers import Adam<br />
from tensorflow.keras.losses import MeanSquaredError<br />
<br />
optimizer = Adam(learning_rate=0.001)<br />
loss_function = MeanSquaredError()<br />
<br />
model.compile(optimizer=optimizer, loss=loss_function)<br />
<br />
# Training loop<br />
for episode in range(1000):<br />
state = env.reset()<br />
done = False<br />
<br />
while not done:<br />
action = model.predict(state)<br />
next_state, reward, done, _ = env.step(action)<br />
<br />
# Update the model<br />
model.fit(state, reward, batch_size=1)<br />
<br />
state = next_state<br />
```<br />
<br />
Finally, once your agent is trained, you can deploy it to interact with the environment. Here's an example of deploying the agent:<br />
<br />
```python<br />
state = env.reset()<br />
done = False<br />
<br />
while not done:<br />
action = model.predict(state)<br />
next_state, reward, done, _ = env.step(action)<br />
<br />
state = next_state<br />
env.render()<br />
```<br />
<br />
And that's it! You now have a basic understanding of how to train and deploy intelligent, self-learning agents using TensorFlow and Python. Happy coding!
#TensorFlow #Reinforcement #Learning #Quick #Start #Guide #running #training #deploying #intelligent #selflearning #agents #Python
Leave a Reply